Nested Loop:
- If a loop placed inside the body of another loop, it’s called a nested loop.
Syntax:
for(int i = 1; i <= 5; i++)
{
for(int j = 1; j <=2; j++)
{
}
}
- for(int i = 1; i <= 5; i++) ==> Outer loop
- for(int j = 1; j <=2; j++) ==> Inner loop
- Above, we created for loop inside the for loop.
- We can create for loop inside the while loop
- And also we can create nested loops with while & do while loop.
- We can use the nested loop in Java to create patterns like full pyramid, half pyramid, inverted pyramid, and so on. (will see more ex in next blog)
Ex 1:
public class Practice2 {
public static void main(String[] args)
{
for (int end = 5; end >= 1; end--)
{
for (int val = 1; val <= end; val++)
{
System.out.print(val + " ");
}
System.out.println();
}
}
}
Output:
1 2 3 4 5
1 2 3 4
1 2 3
1 2
1
- These code derived from below 2 ideas
Idea 1:
public class Practice2 {
public static void main(String[] args)
{
for (int val = 1; val <= 5; val++)
{
System.out.print(val + " ");
}
System.out.println();
for (int val = 1; val <= 4; val++)
{
System.out.print(val + " ");
}
System.out.println();
for (int val = 1; val <= 3; val++)
{
System.out.print(val + " ");
}
System.out.println();
for (int val = 1; val <= 2; val++)
{
System.out.print(val + " ");
}
System.out.println();
for (int val = 1; val <= 1; val++)
{
System.out.print(val + " ");
}
System.out.println();
}
}
Idea 2:
public class Practice2 {
public static void main(String[] args)
{
int end = 5;
while (end >= 1)
{
for (int val = 1; val <= end; val++)
{
System.out.print(val + " ");
}
System.out.println();
end--;
}
}
}
Ex 2:
public class Practice2 {
public static void main(String[] args)
{
for (int end = 1; end <= 5; end++)
{
for (int val = 5; val >= end; val--)
{
System.out.print(val + " ");
}
System.out.println();
}
}
}
Output:
5 4 3 2 1
5 4 3 2
5 4 3
5 4
5
Code Idea 1:
public class Practice2 {
public static void main(String[] args)
{
for (int val = 5; val >= 1; val--)
{
System.out.print(val + " ");
}
System.out.println();
for (int val = 5; val >= 2; val--)
{
System.out.print(val + " ");
}
System.out.println();
for (int val = 5; val >= 3; val--)
{
System.out.print(val + " ");
}
System.out.println();
for (int val = 5; val >= 4; val--)
{
System.out.print(val + " ");
}
System.out.println();
for (int val = 5; val >= 5; val--)
{
System.out.print(val + " ");
}
System.out.println();
}
}
Code Idea 2:
public class Practice2 {
public static void main(String[] args) {
int end = 1;
while (end <= 5)
{
for (int val = 5; val >= end; val--)
{
System.out.print(val + " ");
}
System.out.println();
end++;
}
}
}
Ex 3:
public class Practice2 {
public static void main(String[] args)
{
for (int end = 5; end >= 1; end--)
{
for (int val = end; val >= 1; val--)
{
System.out.print(val + " ");
}
System.out.println();
}
}
}
Output:
5 4 3 2 1
4 3 2 1
3 2 1
2 1
1
Code Idea 1:
public class Practice2 {
public static void main(String[] args)
{
for (int val = 5; val >= 1; val--)
{
System.out.print(val + " ");
}
System.out.println();
for (int val = 4; val >= 1; val--)
{
System.out.print(val + " ");
}
System.out.println();
for (int val = 3; val >= 1; val--)
{
System.out.print(val + " ");
}
System.out.println();
for (int val = 2; val >= 1; val--)
{
System.out.print(val + " ");
}
System.out.println();
for (int val = 1; val >= 1; val--)
{
System.out.print(val + " ");
}
System.out.println();
}
}
Code Idea 2:
public class Practice2 {
public static void main(String[] args)
{
int end = 5;
while (end >= 1)
{
for (int val = end; val >= 1; val--)
{
System.out.print(val + " ");
}
System.out.println();
end--;
}
}
}
Ex 4:
public class Practice2 {
public static void main(String[] args)
{
for (int end = 1; end <= 5; end++)
{
for (int val = end; val <= 5; val++)
{
System.out.print(val + " ");
}
System.out.println();
}
}
}
Output:
1 2 3 4 5
2 3 4 5
3 4 5
4 5
5
Ex 5:
public class Practice2 {
public static void main(String[] args)
{
for (int end = 1; end <= 5; end++)
{
for (int val = end; val <= 5; val++)
{
System.out.print("*" + " ");
}
System.out.println();
}
}
}
Output:
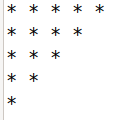
Ex 6:
public class Practice2 {
public static void main(String[] args)
{
for (int end = 5; end >= 1; end--)
{
for (int val = end; val >= 1; val--)
{
System.out.print(end + " ");
}
System.out.println();
}
}
}
Output:
5 5 5 5 5
4 4 4 4
3 3 3
2 2
1
Code Idea 1:
public class Practice2 {
public static void main(String[] args)
{
for (int val = 5; val >= 1; val--)
{
System.out.print(5 + " ");
}
System.out.println();
for (int val = 4; val >= 1; val--)
{
System.out.print(4 + " ");
}
System.out.println();
for (int val = 3; val >= 1; val--)
{
System.out.print(3 + " ");
}
System.out.println();
for (int val = 2; val >= 1; val--)
{
System.out.print(2 + " ");
}
System.out.println();
for (int val = 1; val >= 1; val--)
{
System.out.print(1 + " ");
}
System.out.println();
}
}
Code Idea 2:
public class Practice2 {
public static void main(String[] args)
{
int variable = 5;
while (variable >= 1)
{
for (int val = variable; val >= 1; val--)
{
System.out.print(variable + " ");
}
System.out.println();
variable--;
}
}
}
Ex 7:
public class Practice2 {
public static void main(String[] args)
{
for (int end = 1; end <= 5; end++)
{
for (int val = end; val <= 5; val++)
{
System.out.print(end + " ");
}
System.out.println();
}
}
}
Output:
1 1 1 1 1
2 2 2 2
3 3 3
4 4
5
Code Idea 1:
public class Practice2 {
public static void main(String[] args)
{
for (int val = 1; val <= 5; val++)
{
System.out.print(1 + " ");
}
System.out.println();
for (int val = 2; val <= 5; val++)
{
System.out.print(2 + " ");
}
System.out.println();
for (int val = 3; val <= 5; val++)
{
System.out.print(3 + " ");
}
System.out.println();
for (int val = 4; val <= 5; val++)
{
System.out.print(4 + " ");
}
System.out.println();
for (int val = 5; val <= 5; val++)
{
System.out.print(5 + " ");
}
System.out.println();
}
}
Code Idea 2:
public class Practice2 {
public static void main(String[] args)
{
int variable = 1;
while (variable <= 5)
{
for (int val = variable; val <= 5; val++)
{
System.out.print(variable + " ");
}
System.out.println();
variable++;
}
}
}